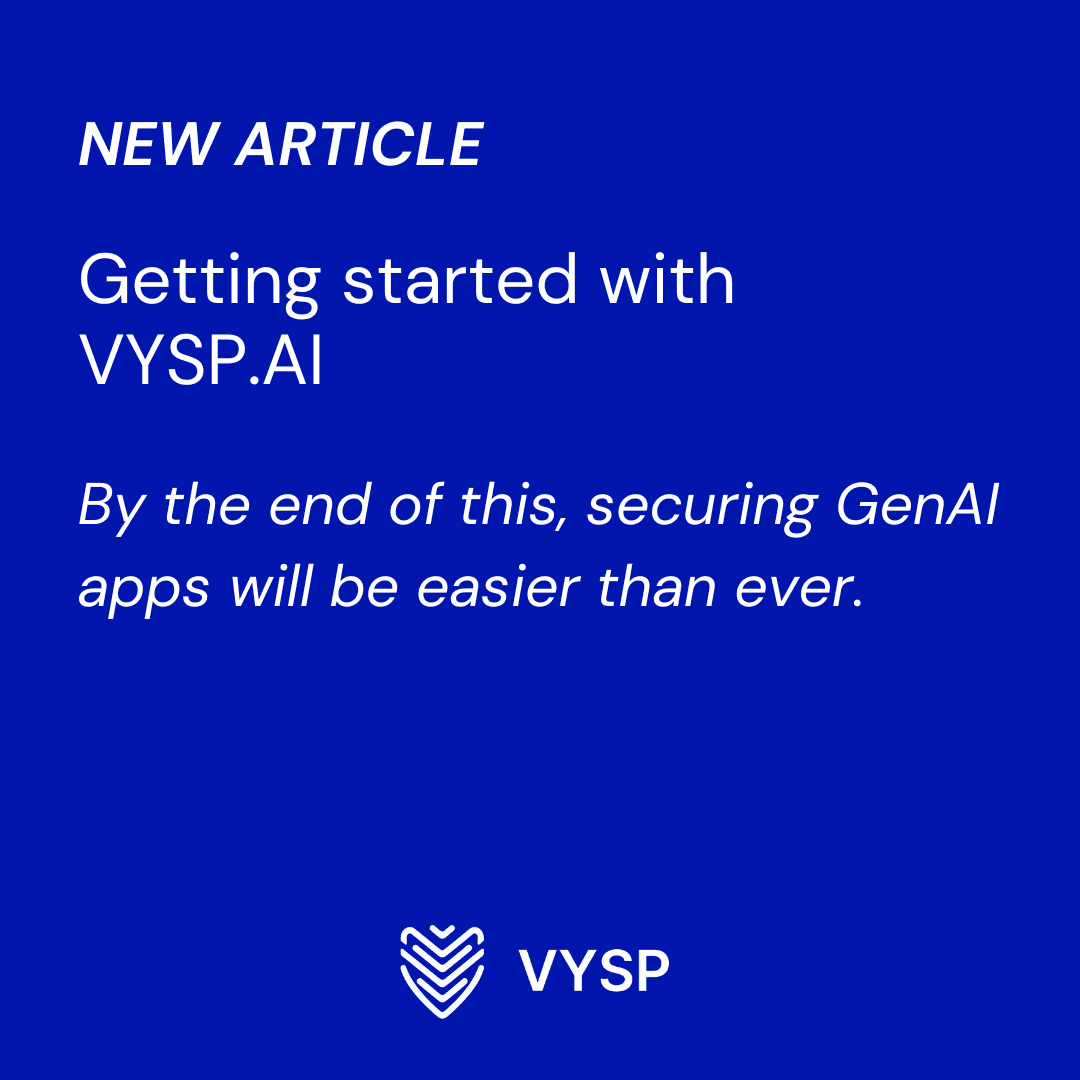
Getting Started with VYSP.AI
Getting Started with VYSP.AI
What is VYSP.AI?
VYSP.AI is a platform that simplifies the configuration and implementation of guardrails for GenAI applications. Guardrails (or what we call Rules) are ML models that perform NLP (and soon, Computer Vision) tasks to keep your applications and users secure. You can easily configure Rules through the web UI and you'd never have to worry about having the processing power to run the machine learning models yourself, because VYSP.AI handles that for you.
Getting Started with VYSP.AI API
Welcome to VYSP.AI, your trusted platform for AI security. This guide will help you get started with our API, walking you through the signup process, creating a gate, and using our Python library to protect a demo ChatGPT application.
Step 1: Sign Up
- Visit our signup page: https://dashboard.vysp.ai/signup.
- Fill in the required details: your name, email, password, and other necessary information.
- Verify your email address through the link sent to your inbox.
- Log in to the dashboard using your newly created credentials.
Step 2: Creating a Gate
- Once logged in, navigate to the Gates section: https://dashboard.vysp.ai/gates.
- Click on "Create New Gate".
- Provide a name for your gate and select the rules you want to apply (e.g., Input Rules, Output Rules).
- Configure the specific settings for each rule as per your requirements.
- Save your gate configuration.
Step 3: Using the Python Library
Installation
First, you need to install the VYSP.AI Python library. You can do this using pip:
pip install vysp-python
Basic Usage
Here is an example of how to perform input and output checks using the library:
import openai
from vysp import VYSPClient
# Initialize the client
client = VYSPClient(tenant_api_key='your_tenant_key', gate_api_key='your_gate_key')
prompt = 'What is VYSP.AI?'
# Perform an input check
input_response = client.check_input(client_ref_user_id='123', prompt=prompt)
print(input_response)
model_output = 'VYSP.AI is a product that allows you to configure AI guardrails easier.'
# Perform an output check
output_response = client.check_output(client_ref_user_id='123', prompt=prompt, model_output=model_output)
print(output_response)
Remember to replace 'your_tenant_key' and 'your_gate_key' with your actual API keys.
Protecting a Demo ChatGPT Application
Here is a step-by-step guide to integrating VYSP.AI with a ChatGPT application using the OpenAI Python library:
1. Import Necessary Libraries
import openai
from vysp import VYSPClient
2. Set Up Your OpenAI API Key and VYSP.AI API Key
Make sure you have your OpenAI API key and VYSP.AI API key ready.
openai.api_key = 'your-openai-api-key'
client = VYSPClient(tenant_api_key='your_tenant_key', gate_api_key='your_gate_key')
3. Create a Function to Send Requests through VYSP.AI
Create a function that sends input and output through the VYSP.AI gate for validation.
def secure_chatgpt_query(prompt):
# Validate input using VYSP.AI
input_response = client.check_input(client_ref_user_id='123', prompt=prompt)
if input_response['is_safe']:
# Send the prompt to OpenAI's ChatGPT
response = openai.Completion.create(
engine="davinci-codex",
prompt=prompt,
max_tokens=150
)
output = response.choices[0].text.strip()
# Validate output using VYSP.AI
output_response = client.check_output(client_ref_user_id='123', prompt=prompt, model_output=output)
if output_response['is_safe']:
return output
else:
return "The response has been flagged as unsafe by VYSP.AI."
else:
return "The input has been flagged as unsafe by VYSP.AI."
4. Test the Integration
You can now test your integration by querying the ChatGPT model:
prompt = "What is the capital of France?"
response = secure_chatgpt_query(prompt)
print(response)
This function first validates the input prompt with VYSP.AI. If the input is deemed safe, it proceeds to send the prompt to OpenAI's ChatGPT. After receiving the response, it validates the output with VYSP.AI. If both input and output are safe, it returns the ChatGPT response; otherwise, it returns an appropriate message.
Conclusion
You have successfully set up your VYSP.AI account, created a gate, and integrated the VYSP.AI security layer into a demo ChatGPT application. For more advanced configurations and features, refer to our detailed documentation.
Feel free to reach out to our support team if you have any questions or need further assistance.